https://github.com/thestonefox/SteamVR_Unity_Toolkit
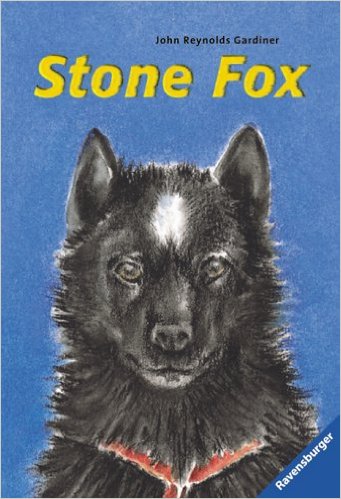
Get HMD Transform from SteamVR Singleton in Unity3D
So maybe I’m too stupid to see this but isn’t there any easy way to get the rotation or position of an TrackedObject from the SteamVR Singleton as an Unity transform? Do I always have to find the steamvr camera in every script?
SteamVR.instance.eyes[0].rot seems to be not changing and SteamVR.instance.hmd.GetEyeToHeadTransform() gives me a matrix, but not a Unity3D, compatible one.
Maybe someone here see what I did not ;).
SteamVR.instance.eyes[0].rot seems to be not changing and SteamVR.instance.hmd.GetEyeToHeadTransform() gives me a matrix, but not a Unity3D, compatible one.
Maybe someone here see what I did not ;).
using UnityEngine; using System.Collections; public class MovementController : MonoBehaviour { private SteamVR_Controller.Device controller { get { return SteamVR_Controller.Input((int)trackedObj.index); } } private SteamVR_TrackedObject trackedObj; public bool dUp = false; public bool dDown = false; public bool dLeft = false; public bool dRight = false; void Start () { trackedObj = GetComponent<SteamVR_TrackedObject>(); } void Update() { if (controller == null) { Debug.Log("Controller not initialized"); return; } if (controller.GetPress(SteamVR_Controller.ButtonMask.Touchpad)) { if (controller.GetAxis(Valve.VR.EVRButtonId.k_EButton_SteamVR_Touchpad).y > 0.5f) { dUp = true; Debug.Log(trackedObj.index + " Movement Dpad Up"); } if (controller.GetAxis(Valve.VR.EVRButtonId.k_EButton_SteamVR_Touchpad).y < -0.5f) { dDown = true; Debug.Log(trackedObj.index + " Movement Dpad Down"); } if (controller.GetAxis(Valve.VR.EVRButtonId.k_EButton_SteamVR_Touchpad).x > 0.5f) { dRight = true; Debug.Log(trackedObj.index + " Movement Dpad Right"); } if (controller.GetAxis(Valve.VR.EVRButtonId.k_EButton_SteamVR_Touchpad).x < -0.5f) { dLeft = true; Debug.Log(trackedObj.index + " Movement Dpad Left"); } } } }
Room-scale VR using Unity3d
Even if you’re new to Unity3d you can get started with a room scale VR experience or port an existing seated or standing VR or 3D application. Specifically, we’ll be targeting the HTC Vive Developer Edition kit using Valve’s SteamVR plugin for Unity3d.
Winds Around Low pressure Systems Turn in the Direction of the Earth’s Rotation and Winds Around High Pressure Systems Turn in the Opposite Direction of the Earth’s Rotation.
using UnityEngine; using System.Collections; using UnityEngine.UI; using Valve.VR; public class GetSteamCtrl_Transform : MonoBehaviour { private Text dbg_Text; public GameObject ctrl1; public GameObject ctrl2; public GameObject reticle_Prefab; private GameObject ret1; private GameObject ret2; private GameObject argosSphere; // Use this for initialization void Start () { dbg_Text = GetComponent<Text>(); argosSphere = GameObject.Find("ArgosSphere"); ret1 = Instantiate(reticle_Prefab); ret2 = Instantiate(reticle_Prefab); } // Update is called once per frame void Update () { dbg_Text.text = "C1 pos = " + ctrl1.transform.position.ToString() + "C2 pos = " + ctrl2.transform.position.ToString(); PositionCursor(ret1, ctrl1.transform); PositionCursor(ret2, ctrl2.transform); } public void PositionCursor(GameObject ret, Transform trans) { float R = argosSphere.GetComponent<ArgosSphere_Indexing>().radius; Vector3 vPC = argosSphere.transform.position - trans.position; Vector3 vBpos = trans.position + Vector3.Dot(vPC, trans.forward) * trans.forward; Vector3 vBC = vBpos - argosSphere.transform.position; float BClen = vBC.magnitude; float BDlen = Mathf.Sqrt(R * R - BClen * BClen); Vector3 D = vBpos + trans.forward * BDlen; ret.transform.position = D; Vector3 vR = D - argosSphere.transform.position; Quaternion rotation = Quaternion.LookRotation(vR.normalized, Vector3.up); ret.transform.rotation = rotation; positionInnerRet(ret, D, trans, vR); } public void positionInnerRet(GameObject ret, Vector3 pos, Transform mPose, Vector3 vR) { Transform inner = ret.transform.GetChild(0); inner.position = pos; Quaternion rotation = Quaternion.LookRotation(vR.normalized, mPose.up); inner.rotation = rotation; } }
lebolay
May 27, 2015 @ 1:12am
aaron.leiby
[developer] May 27, 2015 @ 1:21pm
You will need to convert from OpenVR’s HmdMatrix to Unity’s coordinate space using SteamVR_Utils.RigidTransform the same way SteamVR_TrackedObject does.
pose then will have .pos and .rot for respectively accessing position (Vector3) and rotation (Quaternion). These are relative to the tracking origin, so to convert to world space, also follow SteamVR_TrackedObject’s lead:
where origin is the Transform component of a Unity GameObject that you want things relative to (i.e. the object in your virtual world that you want to correspond to the origin of your physical setup).
aaron.leiby
[developer] May 27, 2015 @ 1:28pm
Also, an easy way to get at the active SteamVR_Camera is to use
This gives you the top-most active SteamVR_Camera. If you only have one SteamVR Camera active in your scene, then it’s pretty obvious which one this is. If you are performing layering (e.g. render a modeled skybox, then a local cockpit on top) this will give you the last layered rendered – the one that renders the cockpit in this example.
Podden
Jun 2, 2015 @ 7:17am