Exercise App
https://www.passion47.com/unity-3d/unity-5-3-using-json-serialization
http://forum.unity3d.com/threads/howto-use-json-in-5-3-guide.373455/
https://sometimesicode.wordpress.com/2015/04/11/unity-serialization-part-1-how-it-works-and-examples/
http://answers.unity3d.com/questions/1171740/android-devices-does-not-read-json-file-but-unity.html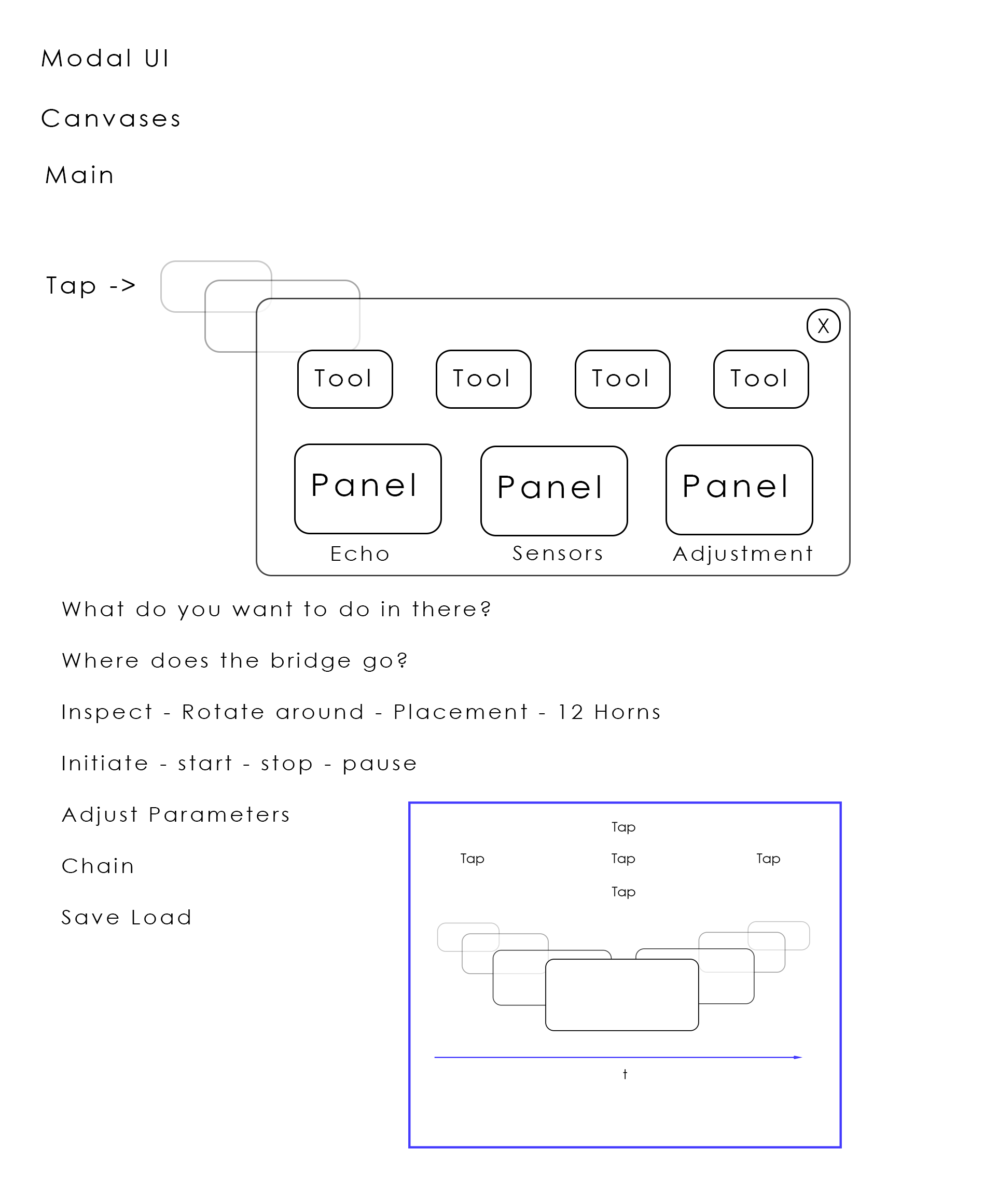
http://sushanta1991.blogspot.com/2014/10/how-to-read-and-write-xml-in-unity.html
Things you should know, First time when you will run the game, there will be no xml present in the directory. So first thing you need to do is save the xml with default values. now you have a xml file where you can save and load data.
what i am doing here is , i have 4 players, i am setting there position randomly , saving their data and then loading there data from xml.
In this function getPath() you can see for Android and Iphone i am using Application.persistentDataPath instead of Application.dataPath that’s because in Iphone and Android you have permission to write files in Application.persistentDataPath only.
using UnityEngine; using System.Collections; using System.Collections.Generic; using System.Xml; using System.Text; using System.IO; public class GameManager : MonoBehaviour { private List<GameObject> Players = new List<GameObject>(); private string path; public string fileName; public Camera myCam; private GameObject previousPlayer; void Start () { GameObject[] allPlayers = new GameObject[GameObject.FindGameObjectsWithTag("Player").Length]; allPlayers = GameObject.FindGameObjectsWithTag("Player"); for(int i = 0; i < allPlayers.Length; i++) { Players.Add(allPlayers[i]); } } public void SetPosition() { for(int i = 0; i < Players.Count; i++) { Players[i].transform.position = new Vector3(Random.Range(-7,7),3,Random.Range(7,42)); } } public void Load() { if(System.IO.File.Exists(getPath())) { path = getPath(); XmlReader reader = XmlReader.Create(path); XmlDocument xmlDoc = new XmlDocument(); xmlDoc.Load(reader); XmlNodeList Data = xmlDoc.GetElementsByTagName("Data"); for(int i = 0; i < Data.Count;i++) { // getting data XmlNode DataChilds = Data.Item(i); // getting all gameObjects stored inside data XmlNodeList allGameObjects = DataChilds.ChildNodes; for(int j = 0; j < allGameObjects.Count ; j++) { XmlNode game_Objects = allGameObjects.Item(j); //Finding the player, if he is present than loading its position and rotation GameObject player = GameObject.Find(game_Objects.Name); if(player) { XmlNodeList GameObjects_Position_Rotation = game_Objects.ChildNodes; //First element have the position stored inside it XmlNode GameObjects_Position = GameObjects_Position_Rotation.Item(0); string[] split_position = GameObjects_Position.InnerText.Split(','); player.transform.position = new Vector3(float.Parse(split_position[0]),float.Parse(split_position[1]),float.Parse(split_position[2])); //Second element have the rotation stored inside it XmlNode GameObjects_Rotation = GameObjects_Position_Rotation.Item(1); string[] split_rotation = GameObjects_Rotation.InnerText.Split(','); player.transform.rotation = new Quaternion(float.Parse(split_rotation[0]),float.Parse(split_rotation[1]),float.Parse(split_rotation[2]),float.Parse(split_rotation[3])); } } } reader.Close(); } } public void Save(){ path = getPath(); XmlDocument xmlDoc = new XmlDocument(); XmlElement elmRoot = xmlDoc.CreateElement("Data"); xmlDoc.AppendChild(elmRoot); for(int i = 0; i < Players.Count; i++) { //Creating an xml element with player name XmlElement Player_Object = xmlDoc.CreateElement(Players[i].name); //Creating an xml element for saving player position XmlElement Player_Position = xmlDoc.CreateElement("Position"); //Combining player position x,y and z value into a single string separeted by commas Player_Position.InnerText = Players[i].transform.position.x+","+Players[i].transform.position.y+","+Players[i].transform.position.z; //Creating an xml element for saving player rotation XmlElement Player_Rotation = xmlDoc.CreateElement("Rotation"); //Combining player rotation x,y,z and w value into a single string separeted by commas Player_Rotation.InnerText = Players[i].transform.rotation.x+","+Players[i].transform.rotation.y+","+Players[i].transform.rotation.z+","+Players[i].transform.rotation.w; Player_Object.AppendChild(Player_Position); Player_Object.AppendChild(Player_Rotation); elmRoot.AppendChild(Player_Object); } StreamWriter outStream = System.IO.File.CreateText(path); xmlDoc.Save(outStream); outStream.Close(); } // Following method is used to retrive the relative path as device platform private string getPath(){ #if UNITY_EDITOR return Application.dataPath +"/Resources/"+fileName; #elif UNITY_ANDROID return Application.persistentDataPath+fileName; #elif UNITY_IPHONE return Application.persistentDataPath+"/"+fileName; #else return Application.dataPath +"/"+ fileName; #endif } }
Tap
Brings up Top Level Menu panel
Panel zoom-fades to gaze location directly in front of user and locks to world space position on transition complete.
(t <1 sec)
Mode is set to Menu.
Tap
On panel dismiss button to return to environ || nav mode || selected tool
——————————————————————————————————————————————————
Tap Tap
In quick succession brings up current tool
Tap
Dismisses
——————————————————————————————————————————————————
Tap – TLM (Top Level Menu)
Panel Placement
Select Panel with
Tap/Hold
Move panel to location desired for panel
Release to place
Tap
On panel dismiss button to close
——————————————————————————————————————————————————-
Head Movements
https://www.cgtrader.com/3d-models/architectural-details/decoration/architectural-kit-bash
tap tap tap